I’m planning on constantly updating and extending this post for the first couple of months that I’m working with Kotlin. I will write down the things that I like about Kotlin, the things I feel are missing or I don’t like, and the things I’m still not sure about. These things may even end up changing their “baskets,” as I’ll be gaining more experience with this language :).
Moreover, discussion and criticism are more than welcome. As I’m just beginning to learn Kotlin, some things may be different in reality than they seem to me now.
The things I like about Kotlin
1. Extension functions
Extension functions are basically more intuitive and less boilerplate util methods. They are declared as functions belonging to some particular class (attention: they aren’t really the member functions of this class, but more on this later), and may be defined anywhere (in some util file or even as a member function of another class).
Yes, you may access class fields (properties in Kotlin) and functions from extension functions without the instance reference. Extension functions are referred to the same way as member functions:Be careful however, because they aren’t member functions and don’t modify the class in any way. What this means is that they could not override the member functions, and the subclasses of the receiver class cannot override these functions as member functions (but they can be overridden as an extension function with a subclass as the receiver).
Apart from extension functions, extension properties may be used also, but with some limitations. As they aren’t really members of an extended class, they may not be initialized, and the getters/setters should be defined explicitly. Basically, they are the same extension functions with shorter notations.
Extension functions are resolved statically, which means that if you have two extension functions for a class and a subclass with the same name, the one declared will always be called (MyClass in this example):
So if you were hoping this would work like magic, it is only sugar, but really sweet sugar, I’ll give it that. Just imagine how many functions you could add to the common classes that you aren’t able to modify. Kotlin already uses some in Collections and Android Extensions. You can add any functions you want to any existing class and call them as they really are inside. Consequently, it appears more logical and drastically reduces the boilerplate code.
Some other extension usage ideas occurred to me that may be combined with other technologies:
First off, the annotation processors (or any code generators) may become more powerful with the help of extensions. It will be quite easy to generate some access methods for existing classes that are using another generated class. We’re planning on trying this approach in our library and will share the results ASAP.
The second usage is the extension of the Observable class for RxKotlin. The creation of new Rx operators has become increasingly easy with the help of extension functions. Just a little example:
What you can achieve with it is limited by your imagination only. How are you using the Kotlin extensions? Feel free to write in the comments or to me directly.2. Functions overloading and default parameters
This is the thing that I really hate about Java. For example, let’s say I want to create a new instance of a fragment, but depending on the place from which I’m creating this instance, I need to pass zero, one, or more arguments. Sometimes those arguments set overlaps, and sometimes they don’t. So this means I need to create more newInstance() methods, pass nulls as arguments (which isn’t readable at all), or use smarter patterns like Builder (which produces even more boilerplate code).
Remember the old lovely query() method from SQLite?
I remember when I was writing my own wrapper for query, I had more than ten overloaded methods. In Kotlin you can forget about overloading in that sense. You can define the function only once with all arguments, define the default values, and call only the ones you need.Moreover, if you need to pass more arguments than just the first one, you can use so-called “named” arguments.
It is really intuitive, readable, and saves you a lot of code.
It is good practice to use named arguments for multi-argument functions even if it is optional, because the programmer may immediately see what is being passed on without going to the function declaration.
To read more of the article continue here…
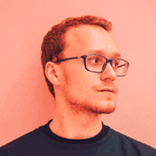