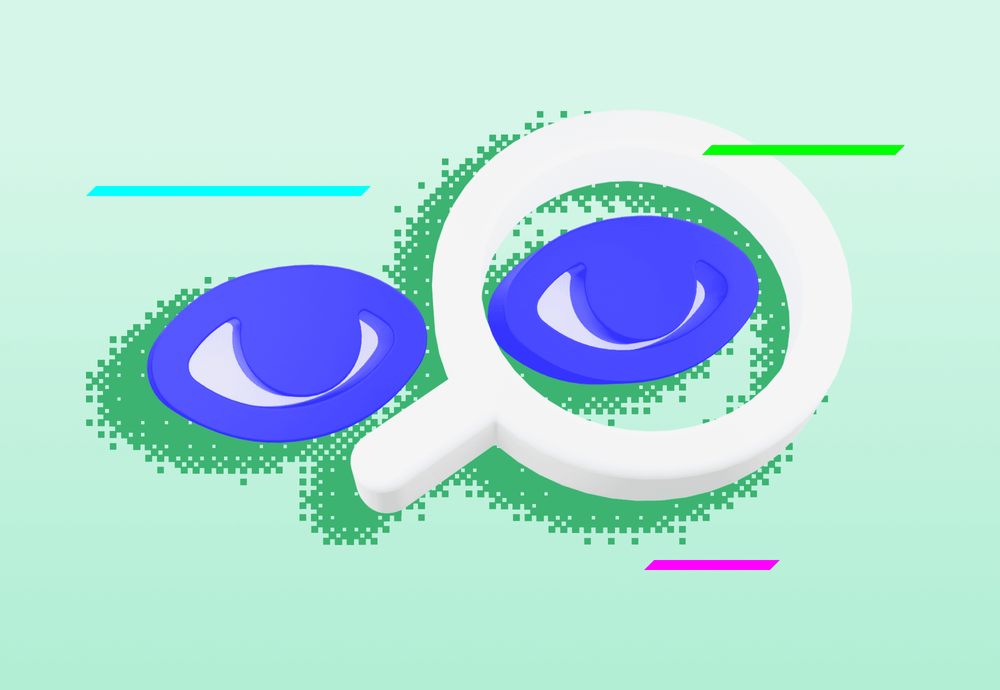
When we first started using Proxyman in 2020, it was a big step up from the Charles Proxy we used previously. Finally, a web-debugging proxy that looked like it was made in the 21st century. Since then, Proxyman has become an essential piece of our toolkit. In this article, I’d like to show you the main use cases for such a tool and how we integrate it into our workflow.
How Proxyman works
Like any other web debugging proxy, Proxyman „sits“ between client apps (mobile apps or web apps in the browser) and a destination server (in most cases, an API). If you send a request from your client app to the server, Proxyman redirects it to the destination server. When the destination server replies, the reply goes through Proxyman back to your client. This man-in-the-middle approach allows Proxyman to not only read the communication but also modify it.
Proxyman: One tool for many use cases
In Ackee, we use Proxyman for many tasks on a day-to-day basis. Here are the scenarios where we find Proxyman the most useful.
Reading network traffic from mobile devices
This is the main feature of every debugging proxy. It might not be as useful for web applications, where all the traffic can be observed via the browser’s built-in DevTools but it’s essential for developing mobile or desktop apps, as they lack built-in developer tools like browsers.
Mocking data
Imagine this situation: you’re developing a new feature in your client app that depends on a specific state sent from your API. Unfortunately, the server implementation is either not ready yet, or it’s just not a viable option for any other reason to get the response in the required format. That’s when intercepting the server response and replacing it with your own mocked data comes in handy. Proxyman provides multiple tools for achieving this:
Breakpoint tool
This is the most straightforward Proxyman tool for this job. First, you must define the matching rules for the resources you want to intercept (you don’t want to modify all the traffic coming in and out of your computer or smartphone). You can match an exact URL path or multiple paths using either a wildcard or RegEx. Moreover, you can select the HTTP method you’re interested in and ignore others. If you are using GraphQL, you can also match requests by specific query names. These matching rules work the same in all the tools mentioned below. Once your configuration is ready, a Proxyman window will pop up whenever you request the defined resource. In the first step, you’ll be able to modify your request. Not just the body but also headers and HTTP method. When you confirm the first step, the request is sent to the destination server. Once Proxyman receives the response, the second breakpoint step is presented to you. In this step, you can modify all the aspects of the response before it is sent to the client app or browser.
This approach is great if you need to alter a request and/or a response. Although it’s very powerful, the pop-up windows can get pretty annoying if you only need to change the response. That’s why there is another tool for the job:
Map Local tool
Map Local lets you adjust just the response, which is often all that's required. For me, this is the feature I utilize the most.
If you want to modify an existing response, just right-click the response you want to mock in the main window and select
Tools -> Map Local
. Any changes to the response you make in the dialog window will be immediately applied to all future requests matching the specified rules. No annoying pop-up windows like when using the breakpoint tool!
Scripting tool
Scripting is the Swiss army knife of the Proxyman’s mocking suite. It has unlimited flexibility thanks to its ability to modify both – requests and responses using JavaScript. The easiest way to get started is to right–click a request and select
Tools -> Scripting
. That way, your matching rules will be pre-filled.Proxyman will give you two basic functions –
onRequest
andonResponse
. Their names are quite self-explanatory and thanks to code examples that Proxyman provides by default, you should be able to start pretty easily:
*/// This func is called if the Request Checkbox is Enabled. You can modify the Request Data here before the request hits to the server*
*/// e.g. Add/Update/Remove: host, scheme, port, path, headers, queries, comment, color and body (json, form, plain-text, base64 encoded string)*
*///*
*/// Use global object \`sharedState\` to share data between Requests/Response from different scripts (e.g. sharedState.data \= "My-Data")*
*///*
async function onRequest(context, url, request) {
*// console.log(request);*
console.log(url);
*// Update or Add new headers*
*// request.headers\["X-New-Headers"\] \= "My-Value";*
*// Update or Add new queries*
*// request.queries\["name"\] \= "Proxyman";*
*// Body*
*// var body \= request.body;*
*// body\["new-key"\] \= "new-value"*
*// request.body \= body;*
*// Done*
return request;
}
*/// This func is called if the Response Checkbox is Enabled. You can modify the Response Data here before it goes to the client*
*/// e.g. Add/Update/Remove: headers, statusCode, comment, color and body (json, plain-text, base64 encoded string)*
*///*
async function onResponse(context, url, request, response) {
*// console.log(response);*
*// Update or Add new headers*
*// response.headers\["Content-Type"\] \= "application/json";*
*// Update status Code*
*// response.statusCode \= 500;*
*// Update Body*
*// var body \= response.body;*
*// body\["new-key"\] \= "Proxyman";*
*// response.body \= body;*
*// Or map a local file as a body*
*// response.bodyFilePath \= "\~/Desktop/myfile.json"*
*// Done*
return response;
}
With JavaScript, the Scripting tool offers near-limitless possibilities for modifying server-client communication. It’s the feature we use if Breakpoint or Map Local tools aren’t flexible enough.
Overview of mocking tools
Here's a quick comparison of Proxyman's mocking tools:
Tool | Breakpoint | Map Local | Scripting |
---|---|---|---|
Modifying requests | ✅ | ❌ | ✅ |
Modifying responses | ✅ | ✅ | ✅ |
Ease of use | Easy | Easy | Advanced |
Annoyance level | Medium | Low | Depends on skill level 🤷♂️ |
Flexibility | Medium | Basic | Limitless |
Proxyman summarized
Proxyman offers a wide range of powerful tools — so many that I couldn’t cover them all in this article! Each tool is designed for a specific use case, but in many situations, multiple tools can achieve the same goal, allowing users to choose what works best for them. At Ackee, Proxyman plays a key role in helping developers and testers with debugging and testing.
If you’d like to see Proxyman in action, check out my talk, where I demonstrate all the mentioned tools in a live demo. The recording is available on YouTube (in Czech).
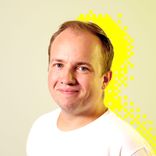